New data centre presence: City Lifeline
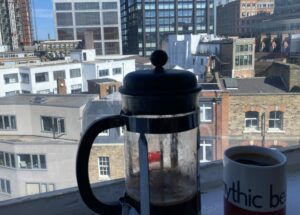
The rest room has a nice view, proper coffee and our branded mugs
In June last year, Digital Realty informed us that they planned to close the Meridian Gate (MER) data centre in 2023. Meridian Gate is our largest presence, so initially this seemed like really bad news. Moving data centres is such a daunting – and expensive – prospect that we’d never really consider it on its own, even if there are long term cost savings or technical benefits. But, once you’re forced to do it, it becomes a rare opportunity to do the kind of upgrades, reorganisation and general tidying that’s so hard to do in racks full of live servers.
Since the announcement we’ve been working hard to figure out not only how to replace the space in MER, but also how to make the most of this chance to configure and kit out new space exactly as we want it.
A key part of the plan is taking on a presence in a new London data centre so that we retain three separate sites in London, and we’re very pleased to announce that our new suite in Redcentric’s City Lifeline (CLL) data centre in Shoreditch is now live, and that our migration out of MER is well underway.
Our CLL presence is connected back to our other two London data centres, Digital Realty’s Sovereign House (SOV) and Equinix LD8 (aka Harbour Exchange/HEX), via a lit fibre ring. The new space allows us to offer dual, redundant 10Gbps to servers, as well as dual redundant power feeds. As with all our data centre space, we have switched PDUs, enabling power to be remotely controlled via our control panel, and remotely accessible serial consoles, so that almost all server issues can be resolved remotely.
If you have services in MER and haven’t already heard from us we’ll be in touch soon to discuss migration plans. We’ve been working hard behind the scenes to minimise disruption to services from the migration out of MER. This includes network upgrades to enable IP portability between MER and CLL so that servers will not need to change IPs during the move and our team are doing a lot of late nights to reduce the impact of any unavoidable disruption.
If you’re interested in taking on new colocated or dedicated servers, please do get in touch as we’ve now got lots of capacity.