Ten years on, Chris Lightfoot looks more prescient than ever
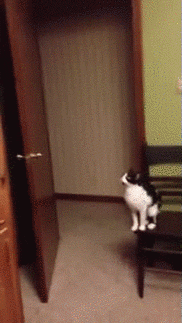
(gif from imgur via gify)
(title shamelessly stolen from Tom Steinberg, MySociety founder from his tribute to Chris, Mythic Beasts founder who died ten years ago).
Lots of people have been excited recently about this script, which allows you to remotely reinstall a Linux system with a different version of Linux by giving you a shell in a ramdisk and letting you reinstall the operating system from there.
Chris did this the hard way. Back in 2005 I remember being asked to code review ‘evil.c’, a script that allocated a lot of RAM (800MB!), compressed a filesystem into it, then uncompressed it back to the disk. On reboot it should come up with Debian instead of FreeBSD that it had earlier. It’s really very important not to swap during this process.
Amazingly it worked, and the first test was on a remote box and it saved us a data centre visit. Here’s the code in its full glory.
#include <sys/types.h> #include <errno.h> #include <fcntl.h> #include <stdio.h> #include <stdlib.h> #include <string.h> #include <unistd.h> #include <zlib.h> #include <sys/mman.h> #include <sys/reboot.h> #define SIZE ((size_t)896058269) #define E(...) fprintf(stderr, __VA_ARGS__) #define die() do { fprintf(stderr, "%s; aborted\n", strerror(errno)); exit(1); } while (0) #define zdie() do { fprintf(stderr, "%s; aborted\n", Z.msg); exit(1); } while (0) int main(void) { unsigned char *buf, *outbuf, *p; int fd; FILE *fp; z_stream Z = {0}; unsigned int nin = 0, nout = 0; E("size = %lu\n", (unsigned long)SIZE); E("open /dev/amrd0... "); if (-1 == (fd = open("/dev/amrd0", O_RDWR | O_DIRECT))) die(); E("done\n"); close(fd); E("allocate file buffer... "); if (!(buf = malloc(SIZE))) die(); E("done\n"); E("allocate write buffer... "); if (!(outbuf = malloc(1024 * 1024))) die(); E("done\n"); E("lock into memory... "); if (-1 == mlockall(MCL_CURRENT | MCL_FUTURE)) die(); E("done\n"); E("open file... "); if (!(fp = fopen("/usr/bitter-first-2100M-of-sda.gz", "rb"))) die(); E("done\n"); E("read file... "); p = buf; while (nin < SIZE) { size_t n; n = fread(p, 1, 262144, fp); if (n == 0) die(); nin += n; p += n; E("\rread file... %.2f%% ", 100 * (float)nin / (float)SIZE); } E("done\n"); fclose(fp); E("zlib version = \"%s\"\n", zlibVersion()); /* Now we need to walk through the buffer decompressing it into the * write buffer, then writing the results to the device. */ E("initialise inflate object... "); Z.next_in = buf; Z.avail_in = SIZE; if (Z_OK != inflateInit2(&Z, 15 + 32)) zdie(); E("done\n"); while (nout < 2100) { int i; size_t N; Z.next_out = outbuf; Z.avail_out = 1024 * 1024; i = inflate(&Z, 0); if (i != Z_OK && i != Z_STREAM_END) zdie(); if (Z.next_out != outbuf + 1024 * 1024) { fprintf(stderr, "\ndidn't get 1MB of output\n"); } /* this is where we'd write the data */ N = 0; p = outbuf; while (N < 1024 * 1024) { ssize_t n; do n = write(fd, p, 1024 * 1024 - N); while (n == -1 && errno == EINTR); if (n == -1) die(); N += n; p += n; } ++nout; fprintf(stderr, "\r%d / 2100 MB", nout); } fprintf(stderr, "\n"); /* this is where we reboot */ reboot(RB_NOSYNC); E("we should have rebooted by now -- probably best to assume we're completely\n" "screwed at this point\n"); return 0; }